Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 정보시스템
- Algorithm
- metasploit
- HTML Injection
- wpscan
- 미터프리터
- algotithm
- study
- SQL Injection
- 취약점
- StringBuilder
- Suninatas
- todo
- 라우터
- java
- stock price
- CSRF
- programmers
- todo List
- ToDoList
- 써니나타스
- 모의해킹
- leetcode
- meterpreter
- 취약점진단
- 웹해킹
- hackerrank
- 모드 설정
- Router
- SQLMap
Archives
- Today
- Total
보안 / 개발 챌린저가 목표
[AlphaGo Study] [LeetCode] [JAVA] 21. Merge Two Sorted Lists 본문
Development/Algorithm
[AlphaGo Study] [LeetCode] [JAVA] 21. Merge Two Sorted Lists
햄미은서 2020. 9. 24. 14:39
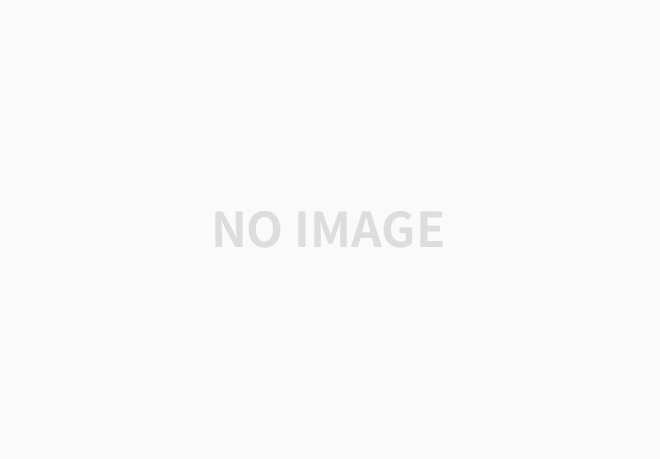
문제 설명
Merge two sorted linked lists and return it as a new sorted list. The new list should be made by splicing together the nodes of the first two lists.
입출력 예
Input | Output | |
l1 | l2 | 1 → 1→ 2 → 3 → 4 → 4 |
1 → 2 → 4 | 1 → 3 →4 |
입출력 예 설명
# 예제
l1의 node에 1 → 2 → 4가 저장되어 있고, l2의 node에 1 →3 → 4 가 저장되어 있다.
이 노드를 합쳐 나온 결과는 1 → 1 → 2 → 3 → 4 → 4 이다.
leetcode.com/problems/merge-two-sorted-lists/
Merge Two Sorted Lists - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
문제를 풀기 전 THINK
- l1의 값과 l2의 값 중 작은 값을 curr_node에 저장한다(작은 순으로 나열해야하기 때문).
- 둘 중 하나가 null이 된다고 해서 정렬을 끝내면 안되기 때문에 if문을 추가한다.
- result_node를 return하면 항상 0값이 나오는데, 필요 없으므로 그 다음 값부터 return한다(return reuslt_node.next).
나의 Solution
public class MergeTwoSortedLists {
public ListNode mergeTwoLists(ListNode l1, ListNode l2) {
ListNode result_node = new ListNode();
// 내가 지정한 값이 head로
ListNode curr_node = result_node;
while(l1 != null && l2 != null) {
if(l1.val < l2.val ) {
curr_node.next = l1;
l1 = l1.next;
} else {
curr_node.next = l2;
l2 = l2.next;
} // if ~ else end
// 노드가 위의 과정을 끝낸 현재와 같도록
curr_node = curr_node.next;
} // while end
// 둘 중 하나가 null이 되어도 다른 하나가 끝날 때 까지 실행
// l1이 null이면 l2를 저장
if(l1 == null) {
curr_node.next = l2;
}
// l2가 null이면 l1을 저장
if(l2 == null) {
curr_node.next = l1;
}
return result_node.next;
}
}
'Development > Algorithm' 카테고리의 다른 글
[AlphaGo Study] [Programmers] [JAVA] 큰 수 만들기 (0) | 2020.10.03 |
---|---|
[AlphaGo Study] [LeetCode] [JAVA] 448. Find All Numbers Disappeared in an Array (0) | 2020.09.28 |
[AlphaGo Study] [LeetCode] [JAVA] 104. Maximum Depth of Binary Tree (0) | 2020.09.22 |
[AlphaGo Study] [HackerRank] [JAVA] Strange Counter (0) | 2020.09.17 |
[AlphaGo Study] [HackerRank] [JAVA] Halloween Sale (0) | 2020.09.14 |
Comments